On employee information management screen, data input via screen is registered and data registered in database is
searched and displayed.
2. Sample Program Description
1. Screen load
'---------------------------------------------------------------------------
Visual WAO SampleCode Employee management system
'
'
' Client operation
'---------------------------------------------------------------------------
' New : 2014/10/24
'---------------------------------------------------------------------------
Public Class EmployeeManagement
' Invoke WAO central
Private waoc As New Wao.WaoInvokeCentral
Private bClose As Boolean
'****************************************************************************
'Screen load
'****************************************************************************
Private Sub EmployeeManagement_Load(sender As Object, e As EventArgs) Handles MyBase.Load
' Start splash screen display
Dim splash = New SplashScreen
splash.Show()
splash.Refresh()
' Update display on splash screen
' (Display splash screen for some time)
'Dim allfiles() As String = IO.Directory.GetFiles("C:\Windows")
Dim allfiles() As String = IO.Directory.GetFiles(System.IO.Directory.GetCurrentDirectory())
Dim curfile As String
For Each curfile In allfiles
splash.ProgressMsg = curfile & " loading……"
Application.DoEvents()
Threading.Thread.Sleep(10)
Next
' End splash screen display
splash.Close()
splash.Dispose()
' Return main form active
Me.Activate()
'##-------------- WAO remote connection setting -----------------------##
'** Invoke remote connection method
If waoc.WaoRemoteOpen("wao", "EmplyoeeManagement", "EmplyoeeManagement_Central") = False Then
MsgBox("Remote connection failed.")
Else
'MSGBOX("Remote connection succeeded.")
End If
'##-------------- WAO remote connection setting -----------------------##
End Sub
I. Employee information management screen startup (client)
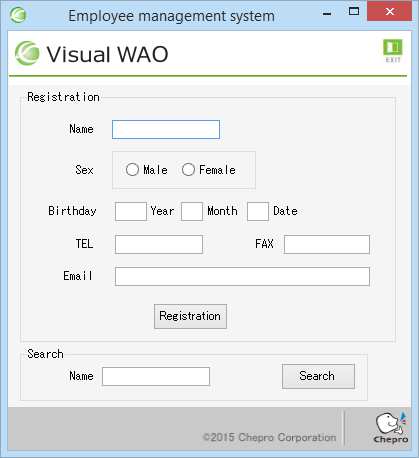
2. Registration button
'****************************************************************************
'Registration button
'****************************************************************************
Private Sub ButtonRegister_Click(sender As Object, e As EventArgs) Handles ButtonRegister.Click
Dim ret%
'Record definition
Dim objEMPLOYEERec As New EMPLOYEERec.EMPLOYEERec
'Parameter array
Dim arrParam As New ArrayList
Try
'##--------------- Central operation invocation Update process -----------------------##
'** Set data for record definition
With objEMPLOYEERec
.username = TextName.Text
.birth = Integer.Parse(TextBirthYYYY.Text & TextBirthMM.Text.PadLeft(2, "0"c) & TextBirthDD.Text.PadLeft(2, "0"c))
.sex = If(RadioButtonMan.Checked, 0, 1)
.tel = TextTel.Text
.fax = TextFax.Text
.mail = TextMail.Text
End With
'Set record definition data in parameter array
arrParam.Add(objEMPLOYEERec)
'** Invoke remote update process method
ret = waoc.WaoUpdate_invokeCentral("update_call", arrParam)
'** Update result decision
If ret = Wao.WaoLib.SYS_NORMAL Then
MsgBox("Registered.", MsgBoxStyle.DefaultButton1, "Employee management system")
Else
MsgBox("Registration failed.", MsgBoxStyle.DefaultButton1, "Employee management system")
End If
'##-------------- Central operation invocation Update process ------------------------##
Catch ex As Exception
MsgBox("Error occurred." & vbNewLine & ex.Message, MsgBoxStyle.DefaultButton1, "Employee management system")
End Try
End Sub
II. Employee information registration process (client)
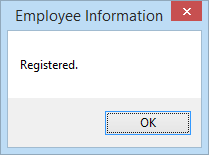
3. Search button
'****************************************************************************
'Search button
'****************************************************************************
Private Sub ButtonSearch_Click(sender As Object, e As EventArgs) Handles ButtonSearch.Click
'##-------------- Central operation invocation Search process -----------------------##
Dim i%, ret%
Dim lin$ = ""
Dim arrParam As New ArrayList
'Parameter setting
arrParam.Add(TextNameSearch.Text)
'** Invoke remote search process method
ret = waoc.WaoRead_invokeCentral("select_call", arrParam, 0)
'Search result decision
If ret = Wao.WaoLib.SYS_NORMAL Then
lin = "Name "
lin = lin & ",Sex"
lin = lin & ",Birthday "
lin = lin & ",TEL "
lin = lin & ",FAX "
lin = lin & ",MAIL"
lin = lin & vbCrLf
'** Obtain search results set for record definition
For i = 0 To waoc.EMPLOYEERecs.Length - 1
lin = lin & waoc.EMPLOYEERecs(i).username.PadRight(10, " ") ' Name
lin = lin & "," & If(waoc.EMPLOYEERecs(i).sex = 0, "Male", "Female") ' Sex
lin = lin & "," & Mid(waoc.EMPLOYEERecs(i).birth.ToString, 1, 4) & "Year" & _
Mid(waoc.EMPLOYEERecs(i).birth.ToString, 5, 2) & "Month" & _
Mid(waoc.EMPLOYEERecs(i).birth.ToString, 7, 2) & "Date" ' Birthday
lin = lin & "," & waoc.EMPLOYEERecs(i).tel.PadRight(13) ' TEL
lin = lin & "," & waoc.EMPLOYEERecs(i).fax.PadRight(13) ' FAX
lin = lin & "," & waoc.EMPLOYEERecs(i).mail ' MAIL
lin = lin & vbCrLf
Next
SearchResult.LabelMessage.Text = lin
SearchResult.ShowDialog()
ElseIf ret = Wao.WaoLib.SYS_NOTFOUND Then
MsgBox("No target record found.", MsgBoxStyle.DefaultButton1, "Employee management system")
End If
'##-------------- Central operation invocation Search process -----------------------##
End Sub
IV. Employee information search process (client)
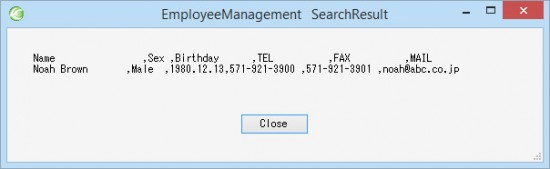
4. Screen close “X” button
'****************************************************************************
'Screen close "X" button
'****************************************************************************
Private Sub EmployeeManagement_FormClosing(ByVal sender As Object, ByVal e As System.Windows.Forms.FormClosingEventArgs) Handles Me.FormClosing
If bClose = True Then
Exit Sub
End If
If WAOClose() <> vbYes Then
e.Cancel = True
End If
End Sub
'****************************************************************************
'Screen close "Exit" button
'****************************************************************************
Private Sub ButtonExit_Click(sender As Object, e As EventArgs) Handles ButtonExit.Click
If WAOClose() = vbYes Then
bClose = True
Me.Close()
End If
End Sub
'****************************************************************************
'Close process
'****************************************************************************
Private Function WAOClose() As Integer
WAOClose = MsgBox("Are you sure you want to close?", MsgBoxStyle.YesNo, "Employee management system")
If WAOClose = vbYes Then
'##-------------- WAO remote disconnection setting -----------------------##
If Not waoc Is Nothing Then
'** Invoke WAO remote disconnection method
waoc.WaoRemoteClose()
waoc.Dispose()
End If
'##-------------- WAO remote disconnection setting -----------------------##
End If
End Function
VI. Employee information management screen end (client)
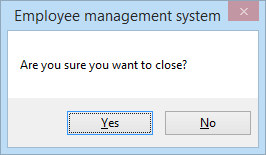
5. Tab transition by Enter key
'****************************************************************************
'Tab transition by Enter key(TextBox)
'****************************************************************************
Private Sub TextBox_Enter(ByVal sender As System.Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles _
TextName.KeyPress, TextBirthYYYY.KeyPress, TextBirthMM.KeyPress, TextBirthDD.KeyPress, _
TextTel.KeyPress, TextFax.KeyPress, TextMail.KeyPress, TextNameSearch.KeyPress
Dim KeyAscii As Short = Asc(e.KeyChar)
Dim ep As TextBox = CType(sender, TextBox)
If e.KeyChar = Microsoft.VisualBasic.ChrW(13) Then
Select Case ep.Name
Case "TextName"
RadioButtonMan.Focus()
Case "RadioButtonMan"
TextBirthYYYY.Focus()
Case "TextBirthYYYY"
TextBirthMM.Focus()
Case "TextBirthMM"
TextBirthDD.Focus()
Case "TextBirthDD"
TextTel.Focus()
Case "TextTel"
TextFax.Focus()
Case "TextFax"
TextMail.Focus()
Case "TextMail"
ButtonRegister.Focus()
Case "TextNameSearch"
ButtonSearch.Focus()
End Select
End If
End Sub
'****************************************************************************
'Tab transition by Enter key (RadioButton)
'****************************************************************************
Private Sub RadioButton_Enter(ByVal sender As System.Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles _
RadioButtonMan.KeyPress, RadioButtonWoman.KeyPress
Dim KeyAscii As Short = Asc(e.KeyChar)
Dim ep As RadioButton = CType(sender, RadioButton)
If e.KeyChar = Microsoft.VisualBasic.ChrW(13) Then
Select Case ep.Name
Case "RadioButtonMan"
TextBirthYYYY.Focus()
Case "RadioButtonWoman"
TextBirthYYYY.Focus()
End Select
End If
End Sub
End Class
6. Switch operation
--------------------------------------------------------------------------
Visual WAO SampleCode Employee management system
'
'
' Central operation
'---------------------------------------------------------------------------
' New : 2014/10/24
'---------------------------------------------------------------------------
Option Explicit On
Option Strict Off
Imports Wao ' WAO class
Imports Wao.WaoLib ' WAO common function
Public Class EmplyoeeManagement_Central
Implements IDisposable
Private DBcom As New Wao.WaoSqlSvDBCom ' WAO SqlServer DataBase class
Private bInit As Boolean = True
Private ErrMsg As String
'****************************************************************************
'Switch operation
'****************************************************************************
Public Function EmplyoeeManagement_Central_SW(ByVal mParameters As ArrayList) As Object
Dim rtn As Wao.RWaoIReturn = New Wao.RWaoIReturn
Dim arr As ArrayList = New ArrayList
Dim ret As Integer
If bInit Then
bInit = False
If DBcom.DBLogin() = False Then
rtn.Result = Wao.RWaoIReturn.RWaoIResult.RTN_STOP
EmplyoeeManagement_Central_SW = rtn
Exit Function
End If
End If
Try
Select Case CType(mParameters(0), String)
Case "select_call"
'** Search process
ret = select_call(CType(mParameters(1), ArrayList))
If ret = SYS_NORMAL Then
arr.Add(DBcom.EMPLOYEERecs.Length) '1st return value: return count (0 counts)
arr.Add(DBcom.EMPLOYEERecs) '2nd return value: searches
rtn.ReturnObj = arr
End If
Case "update_call"
'** Update process
rtn.ReturnObj = update_call(CType(mParameters(1), ArrayList))
Case Else
rtn.Result = Wao.RWaoIReturn.RWaoIResult.RTN_NONE
Exit Try
End Select
If ret = SYS_NOTFOUND Then
arr.Add(0) 'Return count (0 counts)
rtn.ReturnObj = arr
ElseIf ret <> SYS_NORMAL Then
arr.Add(-1) 'Return count (upon error)
arr.Add(ErrMsg) 'Set error message
rtn.ReturnObj = arr
End If
'--------------------------------------------------------------
'rtn.ReturnObj = arr
rtn.Result = Wao.RWaoIReturn.RWaoIResult.RTN_NORMAL
Catch ex As Exception
rtn.Result = Wao.RWaoIReturn.RWaoIResult.RTN_STOP
End Try
EmplyoeeManagement_Central_SW = rtn
End Function
V. Employee information search process (server)
III. Employee information registration process (server)
7. Record search sample
'****************************************************************************
' Record search sample
'****************************************************************************
Public Function select_call(ByVal arr As ArrayList) As Integer
Dim i%, sql$
Dim dbc As New Wao.WaoSqlDbc
select_call = SYS_ERROR
' Memory clear process
If IsNothing(DBcom.EMPLOYEERecs) = False Then
If DBcom.EMPLOYEERecs.Length > 0 Then
For i = 0 To DBcom.EMPLOYEERecs.Length - 1
DBcom.EMPLOYEERecs(i).Dispose()
Next
End If
End If
ReDim DBcom.EMPLOYEERecs(-1)
Try
' Search process
sql = "Select * From EMPLOYEE "
If Trim(CType(arr(0), String)) <> "" Then
sql = sql & "Where USERNAME like '%" & Trim(CType(arr(0), String)) & "%'"
End If
If DBcom.DBReadSQL(dbc, sql) <> SYS_NORMAL Then
select_call = SYS_NOTFOUND
Exit Function
End If
' Obtain search results process
ReDim DBcom.EMPLOYEERecs(dbc.ds.Tables(0).Rows.Count - 1)
For i = 0 To dbc.ds.Tables(0).Rows.Count - 1
DBcom.EMPLOYEERecs(i) = New EMPLOYEERec.EMPLOYEERec(dbc.ds.Tables(0).Rows(i))
If i >= 9 Then
ReDim Preserve DBcom.EMPLOYEERecs(9) '10 counts limit
Exit For
End If
Next i
select_call = SYS_NORMAL
Catch ex As Exception
ErrMsg = ex.Message
Finally
dbc.Dispose()
End Try
End Function
V. Employee information search process (server) *DB process
8. Record registration sample
'*******************************************
'Record registration sample
'*******************************************
Private Function update_call(ByVal arr As ArrayList) As ArrayList
Dim arrReturn As New ArrayList
Dim dts As New DataSet
Dim strSQL As String = ""
Dim arrParam As EMPLOYEERec.EMPLOYEERec = CType(arr(0), EMPLOYEERec.EMPLOYEERec)
'Add new data using screen inputs
strSQL = "INSERT INTO EMPLOYEE (" & vbCrLf
strSQL = strSQL & "USERNAME , " & vbCrLf
strSQL = strSQL & "SEX , " & vbCrLf
strSQL = strSQL & "BIRTH , " & vbCrLf
strSQL = strSQL & "TEL , " & vbCrLf
strSQL = strSQL & "FAX , " & vbCrLf
strSQL = strSQL & "MAIL)" & vbCrLf
strSQL = strSQL & "VALUES (" & vbCrLf
strSQL = strSQL & "'" & arrParam.username & "', " & vbCrLf
strSQL = strSQL & arrParam.sex & ", " & vbCrLf
strSQL = strSQL & arrParam.birth & ", " & vbCrLf
strSQL = strSQL & "'" & arrParam.tel & "', " & vbCrLf
strSQL = strSQL & "'" & arrParam.fax & "', " & vbCrLf
strSQL = strSQL & "'" & arrParam.mail & "'" & vbCrLf
strSQL = strSQL & " )" & vbCrLf
'DB connection, SQL execution
With DBcom
Try
.DBBeginTrans()
.DBExecuteSQL(dts, strSQL)
.DBCommit()
'Normal end
arrReturn.Add(Wao.WaoLib.SYS_NORMAL)
Catch ex As Exception
.DBRollBack()
'Abnormal end
arrReturn.Add(Wao.WaoLib.SYS_ERROR)
Finally
.Dispose()
End Try
End With
Return arrReturn
End Function
Private disposedValue As Boolean = False ' To detect duplicate invocation
' IDisposable
Protected Overridable Sub Dispose(ByVal disposing As Boolean)
If Not Me.disposedValue Then
If disposing Then
' TODO: Release managed resources when explicitly invoked
End If
DBcom.DBClose() 'Database disconnection
' TODO: Release shared unmanaged resources
End If
Me.disposedValue = True
End Sub
#Region " IDisposable Support "
' This code is added by Visual Basic to accurately implement disposable patterns.
Public Sub Dispose() Implements IDisposable.Dispose
' Do not change this code. Clean up code is described in above Dispose(ByVal disposing As Boolean).
Dispose(True)
GC.SuppressFinalize(Me)
End Sub
#End Region
End Class
III. Employee information registration process (server) *DB process
3. Demonstration for Sample Program
1. Development Environment
Development environment: Microsoft Visual Studio 2013
Language: Microsoft Visual Basic
2. Sample Programs
2.1 Folder configuration
When Visual WAO is installed, configuration in “Visual WAO” folder at installation destination drive is as below.
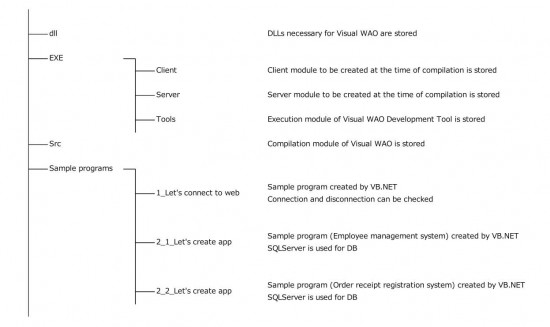
*Details of folder configuration at installation destination can be reviewed in enlarged size by viewing ”InstallationFolderconfiguration.pdf” in “Documents” folder created after unzipping zip file.
3. Let’s Connect to Web with Visual WAO
3.1 Sample program development environment
Language: Microsoft Visual Studio 2013(VB.NET)
3.2 Sample program use
STEP 1: Environment setting (client connection information setting), WAO service startup
STEP 2: Sample program execution (check operation)
STEP 3: Sample program content confirmation
(Debug client/server project with Visual Studio, and check operation)
3.3 STEP 1: Environment setting (client connection information setting), WAO service startup
3.3.1 Start Visual WAO. Main screen below is displayed.
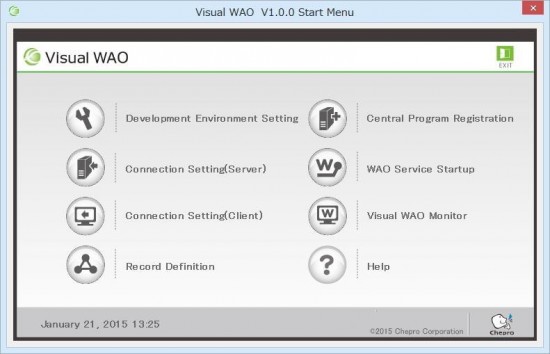
3.3.2 From Visual WAO main screen, press “Connection Setting (Client)” icon.
Connection setting (client) screen is displayed.
Check that “Remote address” is set as “http://localhost:9000/” and press “Update” button.
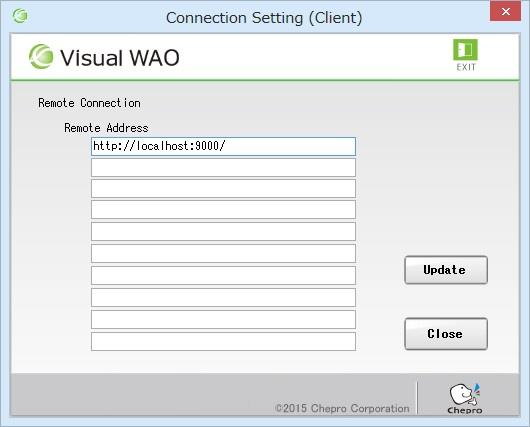
3.3.3 From Visual WAO main screen, press “WAO Service Startup” icon.
Startup triggers display of WAO service icon in task tray.
WAO service icon
3.3.4 To end WAO service, right-click icon and display the menu below.
Select “End.”
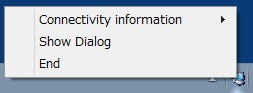
WAO service end menu
3.4 STEP 2: Sample program execution (check operation)
3.4.1 Connection check
Start Sample program screen.
*Execute “C:\Visual WAO\EXE\Client\sample1.exe.”
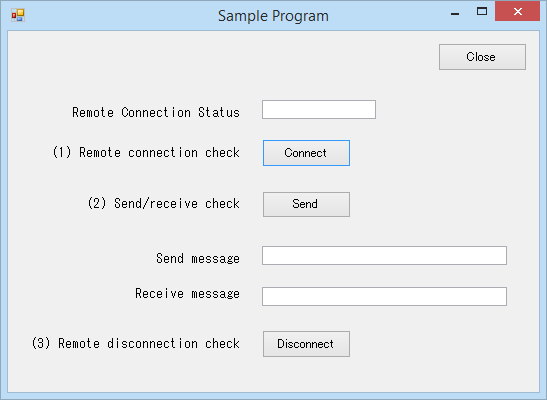
Press “Connect” button for “(1)Remote connection check.”
When successfully connected, message “Remote connection succeeded.” is displayed.
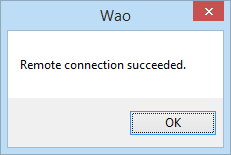
By pressing “OK” button, “Remote connection status” becomes “Connected.”
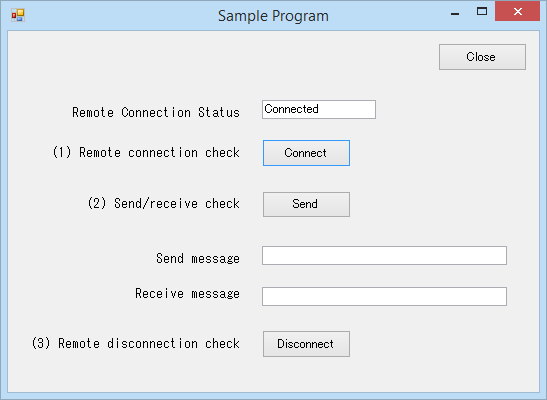
3.4.2 Client-server communication check
In “Send message” item, enter message.
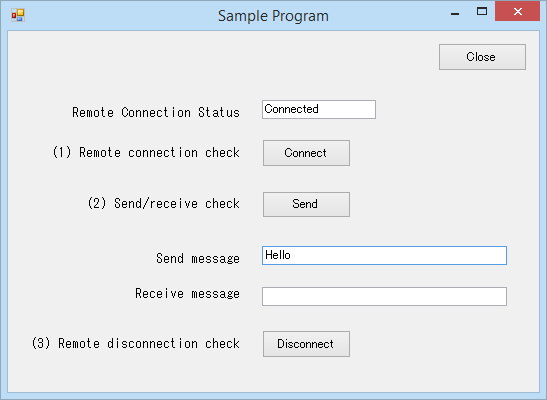
Press “Send” button for “(2)Send/recieve check.”
Message sent is doubled and displayed in “Receive message” item.
In the example below, “Hello” is sent, and “HelloHello” is received.
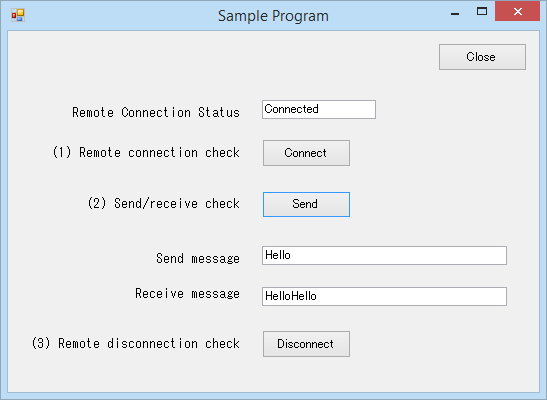
3.4.3 Disconnection check
Press “Disconnect” button for “(3)Remote disconnection check.”
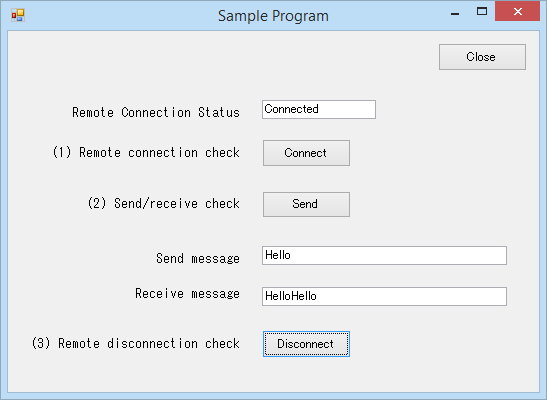
When successfully disconnected, message “Remote connection is disconnected.” is displayed
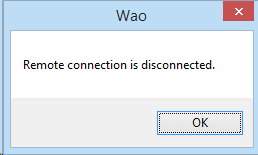
By pressing “OK” button, “Remote connection status” becomes blank.
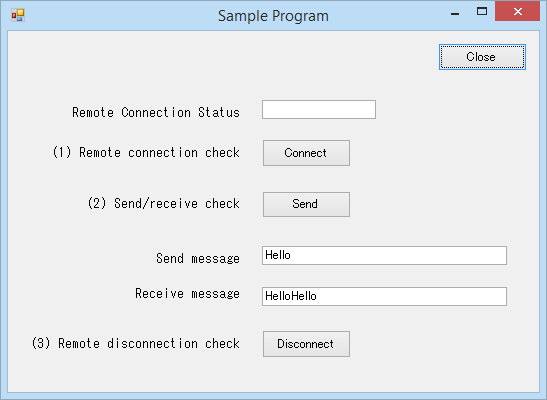
3.4.4 End
To end sample program screen, press “Close” button or “X” button at upper right of screen.
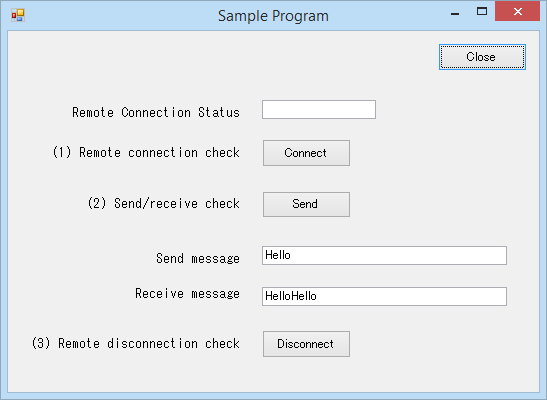
Confirmation message “ Are you sure you want to close sample program screen?” is displayed.
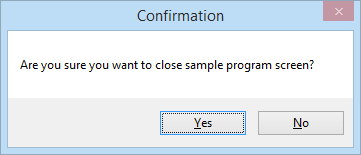
Press “Yes” to end sample program screen. Press “No” to return to sample program screen.
*When connected to server, ending sample program screen simultaneously disconnects remote connection.
3.4.5 Ending WAO service
Right-clicking icon in task tray displays end menu.
Select “End.”
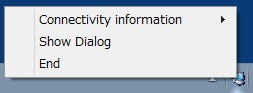
3.5 STEP 3: Sample program content confirmation
(Debug client/server project with Visual Studio, and check operation)
3.5.1 Start sample program project.
Client project “sample1.sln”
Server project “sample1_central.sln”
For each project, refer to the path below.
“C:\Visual WAO\Sample programs \1_Let’s connect to web\sample1\sample1.sln”
“C:\Visual WAO\Sample programs \1_Let’s connect to web\sample1_central\sample1_central.sln”
3.5.2 Execute server project with “Start Debugging.” WAO service is launched.
Execute client project with “Start Debugging.”
Sample program screen (operation checked in STEP 2) is launched.
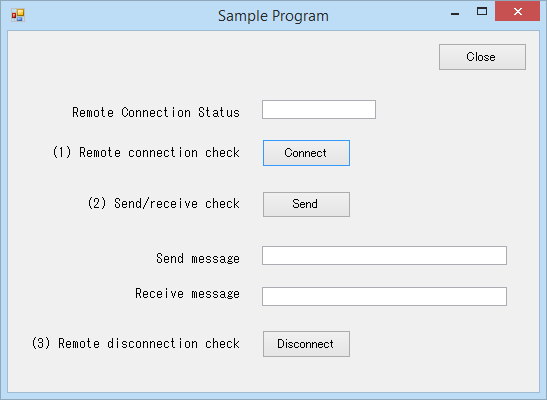
3.5.3 Just as in STEP2, execute in order, starting with “Connect” button for “ (1)Remote connection check.”
It stops at breakpoint set by event at the time of executing client button.
*When resuming execution, WAO function processing from client to server, calling up server project’s class functions, and server program processing can be checked.
4. “Let’s Create App with Visual WAO”
4.1 Sample program development environment
Development language: Microsoft Visual Studio 2013 (VB.NET)
DB: Microsoft SQL Server 2012
STEP 1: Database setting
STEP 2: Sample program execution (check operation)
STEP 3: Sample program content confirmation
(Debug client/server project with Visual Studio, and check operation)
Let’s create app *Employee management system
4.3 STEP 1: Database setting
4.3.1 According to usage environment
Execute “Create_EMPLOYEE.sql” of “C:\Visual WAOSample programs \2_1_Let’s create app\DB\script” folder, and create a table.
4.4 STEP 2: Sample program execution (check operation)
4.4.1 Start Visual WAO. Main screen below is displayed.
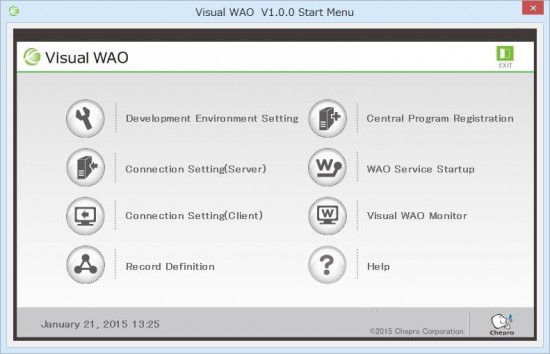
4.4.2 From Visual WAO main screen, press “Connection Setting (Server)” icon.
Connection setting (server) screen is displayed.
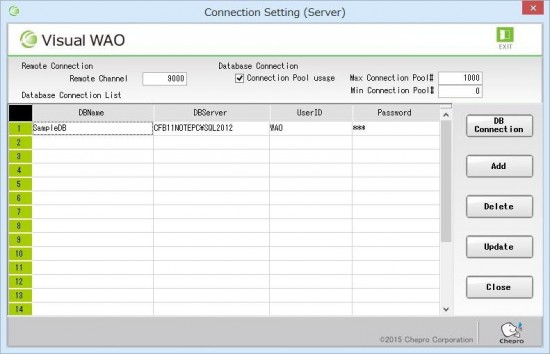
Set “Remote channel” (port number) for remote connection. Default is ”9000.”
Enter database connection settings. Set the following information according to database information set in STEP 1.
(a) DBName: database name
(b) DBServer: database server name
(c) UserID: database login ID
(d) Password: database login password
Press “Update” button. When update is complete, message is displayed.
Settings are registered/updated at “C:\Visual WAO\Exe\Server\Wao.xml.”
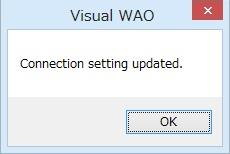
Next, press “DB Connection” button and check connection. Message is displayed.
Confirm successful connection to database.
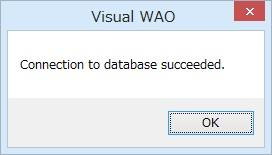
4.4.3 From Visual WAO main screen, press “Connection Setting (Client)” icon.
Connection setting (client) screen is displayed.
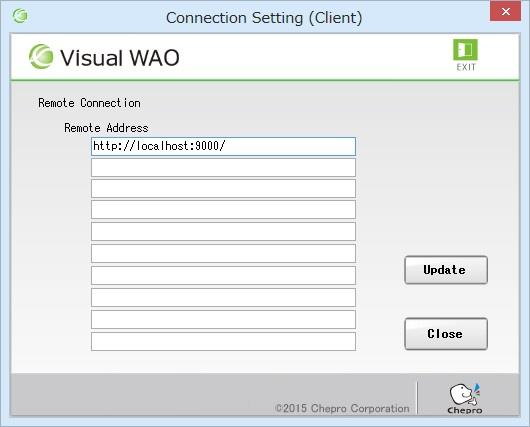
Check that “Remote address” is set as “http://localhost:9000/,” and press “Update” button. Update is executed, and message is displayed.
Settings are registered/updated at “C:\Visual WAO\Exe\Client\RemoteUrls.xml.”
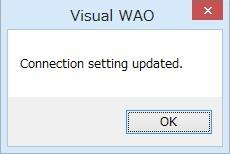
4.4.4 From Visual WAO main screen, press “WAO Service Startup” icon.
Startup triggers display of WAO service icon in task tray.
WAO service icon
To end WAO service, right-click icon, which displays end menu.
Select “End.”
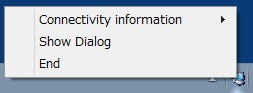
WAO service end menu
4.4.5 Double-click and execute “C:\Visual WAO\EXE\Client\EmployeeManagement.exe.”
Employee management system screen below is displayed.
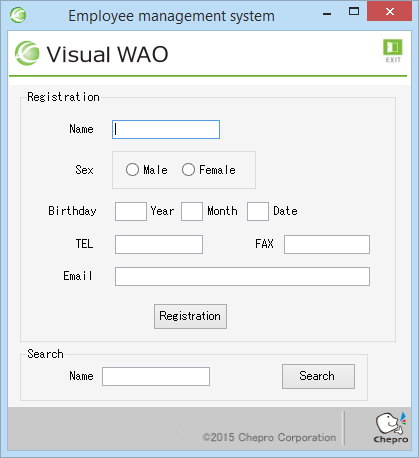
4.5 STEP 3: Sample program content confirmation
(Debug client/server project with Visual Studio, and check operation)
4.5.1 Start sample program project.
Client project “EmployeeManagement.sln”
Server project “EmplyoeeManagement_Central.sln”
For each project, refer to the path below.
“C:\Visual WAO\Sample programs \2_1_Let’s create app\EmployeeManagement\ EmployeeManagement.sln “
“C:\Visual WAO\Sample programs \2_1_Let’s create app\EmployeeManagement\ EmplyoeeManagement_Central.sln “
4.5.2 Execute server project with “Start Debugging.” WAO service is launched.
Execute client project with “Start Debugging.”
Sample program screen (operation checked in STEP 2) is launched.
4.5.3 Connection to server is done by event from client, and logic processing is executed.
By setting breakpoint with client program and server program, client and server operation can be checked.
*Details of “Employee management system” of 2_1_Let’s create app can be reviewed in the following files in “Documents” folder created after unzipping zip file.
– EmployeeManagement_System Overview.pdf
– EmployeeManagement_Program Description.pdf
Let’s create app *Order receipt registration system
4.6 STEP 1: Database setting
4.6.1 According to your environment, set database from “C:\Visual WAOSample programs \2_2_Let’s create app\DB.”
Setting can be selected from the three patterns below. Set according to usage environment.
a) Script: Execute script to create table, and paste sample data to created table.
b) For detach: Detach MDF file with SQLServer tool.
c) Backup file: Restore backup file with SQLServer tool.
4.7 STEP 2: Sample program execution (check operation)
4.7.1 Start Visual WAO. Main screen below is displayed.
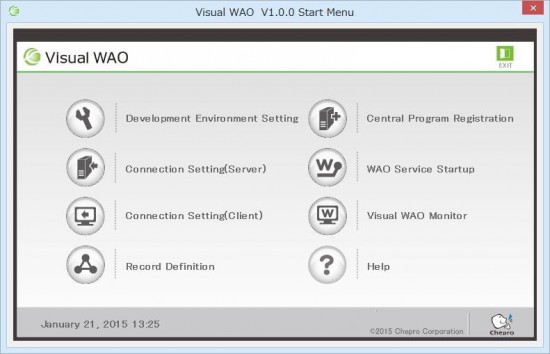
4.7.2 From Visual WAO main screen, press “Connection Setting (Server)” icon.
Connection setting (server) screen is displayed.
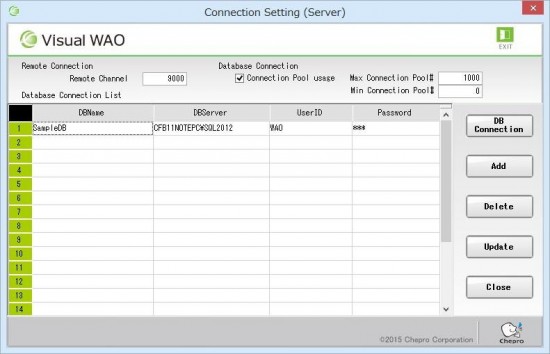
Set “Remote channel “ (port number) for remote connection. Default is “9000.”
Enter database connection settings. Set the following information according to database information set in STEP 1.
(a) DBName: database name
(b) DBServer: database server name
(c) UserID: database login ID
(d) Password: database login password
Press “Update” button. When update is complete, message is displayed.
Settings are registered/updated at “C:\Visual WAO\Exe\Server\Wao.xml.”
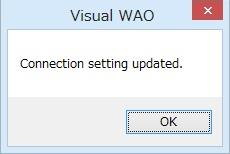
・Next, press “DB Connection” button and check connection. Message is displayed.
Confirm successful connection to database.
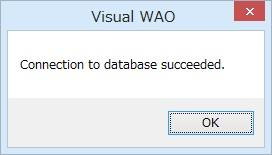
4.7.3 From Visual WAO main screen, press “Connection Setting (Client)” icon.
Connection setting (client) screen is displayed.
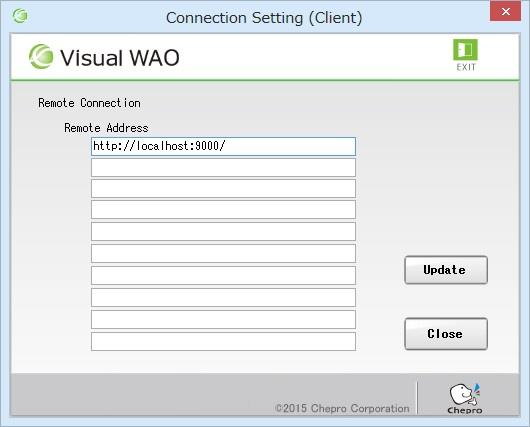
・Confirm “Remote address” is set as “http://localhost:9000/,” and press “Update” button. Update process is executed, and message is displayed.
Settings are registered/updated at “C:\Visual WAO\Exe\Client\RemoteUrls.xml.”
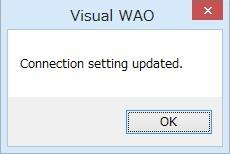
4.7.4 From Visual WAO main screen, press “WAO Service Startup”icon.
Startup triggers display of WAO service icon in task tray.
WAO service icon
To end WAO service, right-click icon, which displays end menu.
Select “End.”
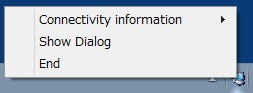
WAO service end menu
4.7.5 Double-click and execute “C:\Visual WAO\EXE\Client\WAOSample_Client.exe.”
Order receipt registration screen below is displayed.
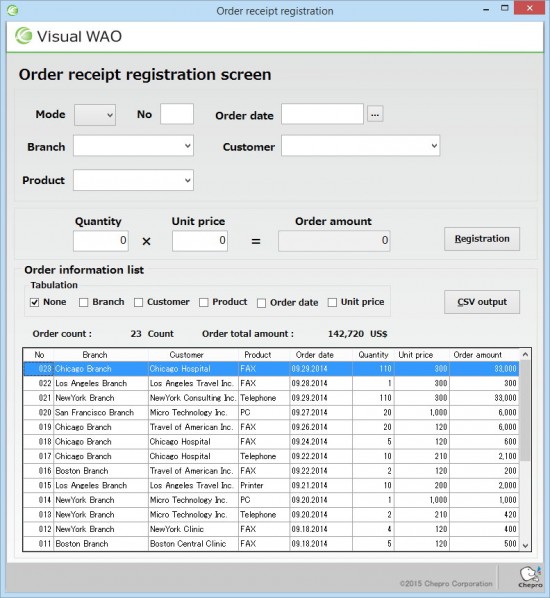
4.8 STEP 3: Sample program content confirmation
(Debug client/server project with Visual Studio, and check operation)
4.8.1 Start sample program project.
Client project “WAOSample.sln”
Server project “WAOSample_Central.sln”
For each project, refer to the path below.
“C:\Visual WAO\Sample programs \2_2_Let’s create app\WAO_Client\WAOSample.sln”
“C:\Visual WAO\Sample programs \2_2_Let’s create app\WAO_Server\WAOSample_Central.sln”
4.8.2 Execute server project with “Start Debugging.” WAO service is launched.
Execute client project with “Start Debugging.”
Sample program screen (operation checked in STEP 2) is launched.
4.8.3 Connection to server is done with event from client, and logic processing is executed.
By setting breakpoint with client program and server program, client and server operation can be checked.
5. Function Specifications
5.1 Client application function
Remote connection method
Method name |
Wao.WaoInvokeCentral.RemoteOpen |
Arguments |
ByVal _loginid$, Set if login information is managed ByVal _apname$, Local program name ByVal _startcall$ Launch string (function ID name) |
Return values |
Boolean true: Connect successfully, false: Connect failed |
Remarks |
- For each application, remote connection must be done only once at the beginning.
- When connection fails or disconnects in the middle, whether or not to reconnect can be checked.
- For launch string, set unique string within overall development system.
|
Remote disconnection method
Method name |
Wao.WaoInvokeCentral.WaoRemoteClose |
Arguments |
NA |
Return values |
Boolean true: successful, false: failed |
Remarks |
- When remotely connected, make sure to execute disconnection method at the end.
|
Remote search processing method (to receive information record)
Method name |
Wao.WaoInvokeCentral.WaoRead_invokeCentral |
Arguments |
ByVal func$, function ID name ByVal keyrec As Object, search parameters ByVal keyid As Integer search number ByRef recs As Object acquired information record (can be omitted) |
Return values |
Integer 0: normal, 1: failed, -1: no record |
Remarks |
- For function ID, use unique string within overall development system.
- For search parameters, designate string or serialized class object.
- Freely use search number with application.
- For acquired information record, designate string or serialized class object.
- When these arguments are omitted, acquired information record is stored in “Wao.WaoInvokeCentral.[recordID]s().”
*To omit acquired information record, make sure to execute “DLL Update” in record definition of Visual WAO.
|
Remote search processing method (to receive information record)
Method name |
Wao.WaoInvokeCentral.WaoReadA_invokeCentral |
Arguments |
- ByVal func$, function ID name
- ByVal keys As ArrayList, search parameter
- ByVal keyid As Integer search number
- ByRef recs As Object acquired information record (can be omitted)
|
Return values |
Integer 0: normal, 1: failed, -1: no record |
Remarks |
- For function ID, use unique string within overall development system.
- For search parameters, designate string or serialized class object.
- Freely use search number with application.
- For acquired information record, designate string or serialized class object.
- When these arguments are omitted, acquired information record is stored in “Wao.WaoInvokeCentral.[recordID]s().”
*To omit acquired information record, make sure to execute “DLL Update” in record definition of Visual WAO.
|
Remote updating processing method
Method name |
Wao.WaoInvokeCentral.WaoUpdate_invokeCentral |
Arguments |
ByVal func$, function ID name ByVal prm1 As Object, Processing parameters |
Return values |
Integer 0: normal, 1: failed, -1: no record |
Remarks |
For function ID, use unique string within overall development system. For processing parameters, designate character string or serialized class object. |
Remote updating processing method
Method name |
Wao.WaoInvokeCentral.WaoUpdateA_invokeCentral |
Arguments |
ByVal func$, function ID name ByVal param As ArrayList Processing parameters |
Return values |
Integer 0: normal, 1: failed, -1: no record |
Remarks |
For function ID, use unique string within overall development system. For processing parameters, designate character string or serialized class object. |
5.2 Server application function
Remote invocation method
Method name |
Class name(Assembly name) + ‘_SW’ |
Arguments |
ByVal mParameters As ArrayList Passing argument from remote |
Return values |
Wao.RWaoIReturn Return information class |
Remarks |
|